SMPP Lua Agent
Introduction
The SMPPLuaAgent is an asynchronous helper for Lua scripts running within the LogicApp. It is used for sending out SMPP client requests.
- The SMPP Agent sends outbound SMPP requests. It is part of the core N2SVCD framework.
- The SMPP Service handles inbound SMPP requests. It is part of the separate N2TTG product.
The SMPPLuaAgent communicates with one or more instances of the SMPPApp which can be used to communicate with one or more external SMPP entities.
The SMPPLuaAgent communicates with the SMPPApp using the SMPP-C-… messages.
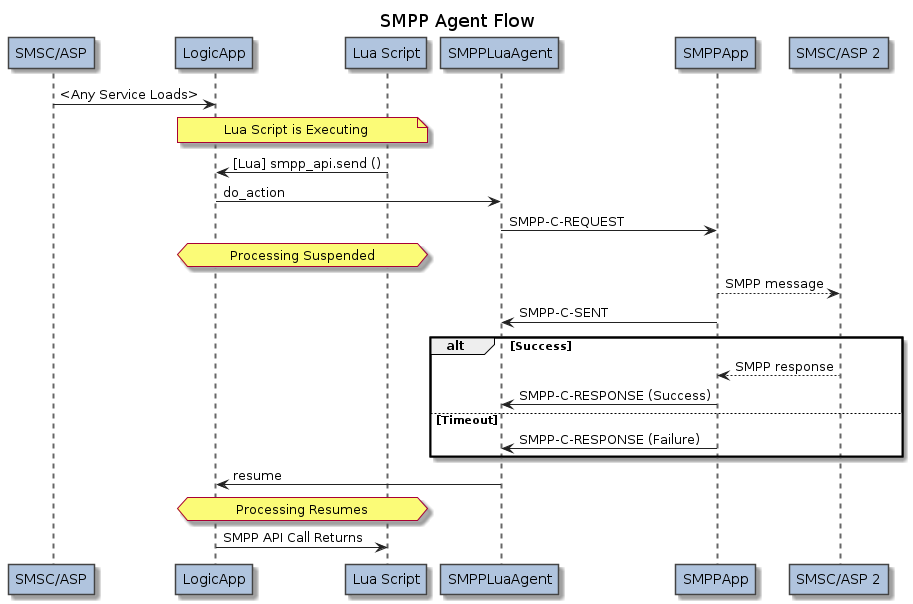
SMPP Agent API methods are accessed via the “n2.n2svcd.smpp_agent” module:
local smpp_agent = require "n2.n2svcd.smpp_agent"
Configuring SMPPLuaAgent
The SMPPLuaAgent is configured within a LogicApp.
<?xml version="1.0" encoding="utf-8"?>
<n2svcd>
...
<applications>
...
<application name="Logic" module="LogicApp">
<include>
<lib>../apps/logic/lib</lib>
</include>
<parameters>
...
<parameter name="default_smpp_app_name" value="SMPP-CLIENT"/>
</parameters>
<config>
<services>
...
</services>
<agents>
<agent module="SMPPApp::SMPPLuaAgent" libs="../apps/smpp/lib"/>
</agents>
</config>
</application>
...
</application>
...
</n2svcd>
Under normal installation, the following agent
attributes apply:
Parameter Name | Type | XML Type | Description |
---|---|---|---|
module
|
SMPPApp::SMPPLuaAgent
|
Attribute | [Required] The module name containing the Lua Agent code. |
libs
|
../apps/smpp/lib
|
Attribute |
Location of the module for SMPPLuaAgent.
|
In addition, the SMPPLuaAgent must be configured with the name of the SMPPApp with which
it will communicate. This is configured within the parameters
of the containing
LogicApp
.
Parameter Name | Type | XML Type | Description |
---|---|---|---|
parameters
|
Array | Element |
Array of name = value Parameters for this Application instance.
|
.default_smpp_app_name
|
String | Attribute | Default name for the SMPPApp with which SMPPLuaAgent will communicate. |
.smpp_app_name_
|
String | Attribute |
Use this format when SMPPLuaAgent will communicate with more than one SMPPApp .(Default = SMPPLuaAgent uses only the default route/SMPP end-point). |
The SMPPLuaAgent API
All methods may raise a Lua Error in the case of exception, including:
- Invalid input parameters supplied by Lua script.
- Unexpected results structure returned from SMPPApp.
- Processing error occurred at SMPPApp.
- Timeout occurred at SMPPApp.
The SMPPLuaAgent API can be loaded as follows.
local smpp_agent = require "n2.n2svcd.smpp_agent"
.request_response [Synchronous]
Send a new SMPP message to the network. The Lua service logic will suspend until the request is acknowledged by the far-end.
The request_response
method takes the following parameters.
Parameter | Type | Description |
---|---|---|
pdu
|
String |
The SMPP message type to send. Must be either deliver_sm or submit_sm .
|
fields
|
Object |
The fields to send for the message. The possible fields are as shown in the
fields parameter of the SMPP-C-REQUEST message.
|
This method returns a single parameter as a Lua table containing the contents of the received SMPP-C-RESPONSE message.
[Fragment] Example (Submit SM):
...
smpp_agent.request_response ("submit_sm", { source_addr = "123", destination_addr = "641234567" })
...
.send_request [Asynchronous]
Send a new SMPP message to the network.
The Lua service logic will return success if the message was accepted by the N2SVCD subsystem and queued for delivery over a link with a SMPP far-end. This method will error if the message could not be queued.
The send_request
method takes the following parameters.
Parameter | Type | Description |
---|---|---|
pdu
|
String |
The SMPP message type to send. Must be either deliver_sm or submit_sm .
|
fields
|
Object |
The fields to send for the message. The possible fields are as shown in the
fields parameter of the SMPP-C-REQUEST message.
|
This method returns no information. On error, a Lua error()
call is made, so
pcall()
should be used to catch errors from this method.
[Fragment] Example (Submit SM):
...
smpp_agent.send_request ("submit_sm", { source_addr = "123", destination_addr = "641234567" })
...
.expect_response [Synchronous]
Suspend until a far-end response is received (from the far-end SMPP server)
for the last sent request, or a timeout or error occurs. Technically this
suspends until a SMPP-C-RESPONSE
message is received by the Lua instance from an
n2svcd SMPP application. If send_request()
is used twice in succession, then
this method will wait for a response of either.
The expect_response
method takes no parameters.
This method returns a single parameter as a Lua table containing the contents of the received SMPP-C-RESPONSE message.
[Fragment] Example):
...
local ok, smpp_response = pcall(function () return smpp_agent.expect_response() end)
...
.response_ready [Asynchronous]
Checks whether a far-end response has been received (from the far-end SMPP
server) for the last sent request, or a timeout or other error occurs.
Technically this checks for any SMPP-C-RESPONSE
message to have been received
by the Lua instance engine, from another n2svcd SMPP application.
The response_ready()
method takes no parameters.
This method returns true
or false
. If true
, a call to expect_response()
will
return immediately if called.
Note that n2svcd.progress()
or another action that waits on IPC message queue
processing should be called prior to calling this.
[Fragment] Example:
...
local progress = n2svcd.progress (1)
if (progress[smpp_agent.AGENT_KEY]) then
local have_smpp_response = smpp_agent.response_ready()
if have_smpp_response then
local ok, smpp_response = pcall(function () return smpp_agent.expect_response() end)
...
end
end
.submit and .deliver [Asynchronous]
Sends a new SMPP submit_sm
or deliver_sm
message to the network as per the send
method.
The submit
and deliver
methods take the following parameters.
Parameter | Type | Description |
---|---|---|
source_addr
|
String | Address of the SME from which the message originated. |
destination_addr
|
Object | Address of the SME from which the message originated. |
short_message_text
|
String | The non-encoded message text. This will be automatically encoded when the message is constructed. |
This method has the same response as the request_response
method.
[Fragment] Example (Submit, Deliver):
...
smpp_agent.submit ("123", "641234567", "Hello, world!")
smpp_agent.deliver ("641234567", "123", "Hello, world!")
...